Create a pipeline
A pipeline can be created from a solution template in the app or through the API.
A pipeline have following properties:
- a logical key identifier of maximum 32 char (required)
- a link to a template (required)
- a set of variables (optional)
- a list of steps (required)
Using Python
To create or update a pipeline you can use a JSON definition or define it from code directly. Below here is a sample script to create a pipeline and its equivalent JSON definition
import wizata_dsapi
pipeline = wizata_dsapi.Pipeline(
key="my_pipeline",
template_id=uuid.UUID('639e7537-0d80-4056-9670-06a63870d386'),
variables={
"var1": "string",
"from": "relative",
"to": "relative"
}
)
pipeline.add_query(
request=wizata_dsapi.Request(
datapoints=['dp1','dp2','dp3'],
interval=60000,
agg_method='mean',
start="@from",
end="@to"
),
df_name="query_output"
)
pipeline.add_transformation(
script="my_script",
input_df_names=['query_output'],
output_df_names=['transform_data']
)
pipeline.add_model(
config=wizata_dsapi.MLModelConfig(
model_key='my_model_key',
train_script='linear_reg_sc',
features=['dp1', 'dp2'],
target_feat='dp3',
),
input_df='transform_data',
output_df='predicted_data'
)
pipeline.add_writer(
config=wizata_dsapi.WriteConfig(
datapoints={
'predicted_dp': 'dp_output'
}
),
input_df='predicted_data'
)
pipeline.add_plot(
df_name='predicted_data',
script='describe_df'
)
wizata_dsapi.api().upsert_pipeline(pipeline)
{
"key": "my_pipeline",
"templateId": "639e7537-0d80-4056-9670-06a63870d386",
"steps": [
{
"type": "query",
"config": {
"equipments_list": [
{
"id": null,
"datapoints": [
"dp1",
"dp2",
"dp3"
],
"shift": "0s"
}
],
"timeframe": {
"start": "@from",
"end": "@to"
},
"aggregations": {
"agg_method": "mean",
"interval": 60000
},
"filters": {},
"options": {}
},
"inputs": [],
"outputs": [
{
"dataframe": "query_output"
}
]
},
{
"type": "script",
"config": {
"function": "my_script"
},
"inputs": [
{
"dataframe": "query_output"
}
],
"outputs": [
{
"dataframe": "transform_data"
}
]
},
{
"type": "model",
"config": {
"train_script": "linear_reg_sc",
"train_test_split_pct": 1,
"train_test_split_type": "ignore",
"target_feat": "dp3",
"features": [
"dp1",
"dp2"
],
"model_key": "my_model_key",
"output_property": "result",
"function": "predict"
},
"inputs": [
{
"dataframe": "transform_data"
}
],
"outputs": [
{
"dataframe": "predicted_data"
}
]
},
{
"type": "write",
"config": {
"datapoints": {
"predicted_dp": "dp_output"
}
},
"inputs": [
{
"dataframe": "predicted_data"
}
],
"outputs": []
}
],
"variables": {
"var1": "string",
"from": "relative",
"to": "relative"
}
}
You can therefore alternatively create or update your pipeline directly from a JSON.
import wizata_dsapi
import json
with open('your_pipeline.json', 'r') as f:
pipeline_dict = json.load(f)
pipeline = wizata_dsapi.Pipeline()
pipeline.from_json(pipeline_dict)
wizata_dsapi.api().upsert_pipeline(pipeline)
Using UI
Navigate to AI Lab > Pipelines and click on 'Add' to create a new pipeline.
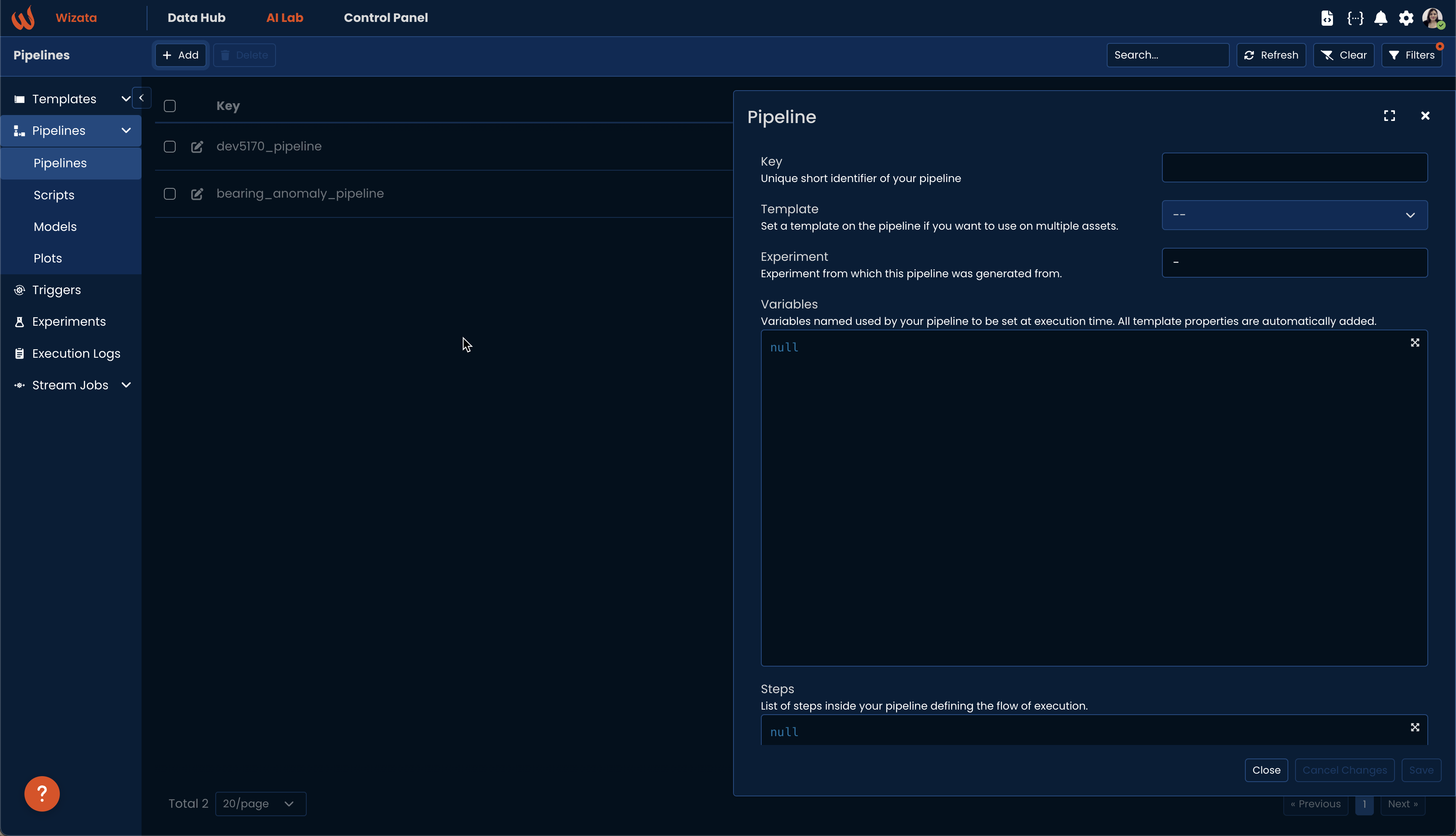
Updated 5 months ago