Template
A Template is a user defined concept similar to a class, that groups common properties (e.g. properties of different twin items).
Templates allows you to bind together set of datapoints and properties common to your solution. You can typically define a re-usable schema in order to scale easily your solutions across all similar templates.
Creating a Template
To start registering a template inside the platform, you need to define an unique key identifier, a name and its properties. Properties of a template are typically datapoint (timeseries) or variable (string, integer and float).
For example: A “my_example_factory_template” might have as datapoint, Bearing1, Bearing2 and Bearing_anomaly and as variable threshold and as JSON Bearing_properties
Using the Python Toolkit, you can register a template using the following logic:
wizata_dsapi.api().upsert_template(
key="my_example_factory_template",
name="My Example Factory Template"
)
wizata_dsapi.api().add_template_property(
template='my_example_factory_template',
property_name='Bearing1'
)
wizata_dsapi.api().add_template_property(
template='my_example_factory_template',
property_name='Bearing2'
)
wizata_dsapi.api().add_template_property(
template='my_example_factory_template',
property_name='Bearing3'
)
wizata_dsapi.api().add_template_property(
template='my_example_factory_template',
property_name='Bearing4',
property_type='datapoint'
)
wizata_dsapi.api().add_template_property(
template='my_example_factory_template',
property_name='bearing_anomaly',
property_type='datapoint'
)
wizata_dsapi.api().add_template_property(
template='my_example_factory_template',
property_name='Threshold',
property_type='float'
)
wizata_dsapi.api().add_template_property(
template='my_example_factory_template',
property_name='Bearing_properties',
property_type='json'
)
property_type
is by default datapoint and therefore not required.
We also have technical documentation for the Template object in our Python SDK documentation.
Or parallelly, using the UI, clicking on Add and completing the form:
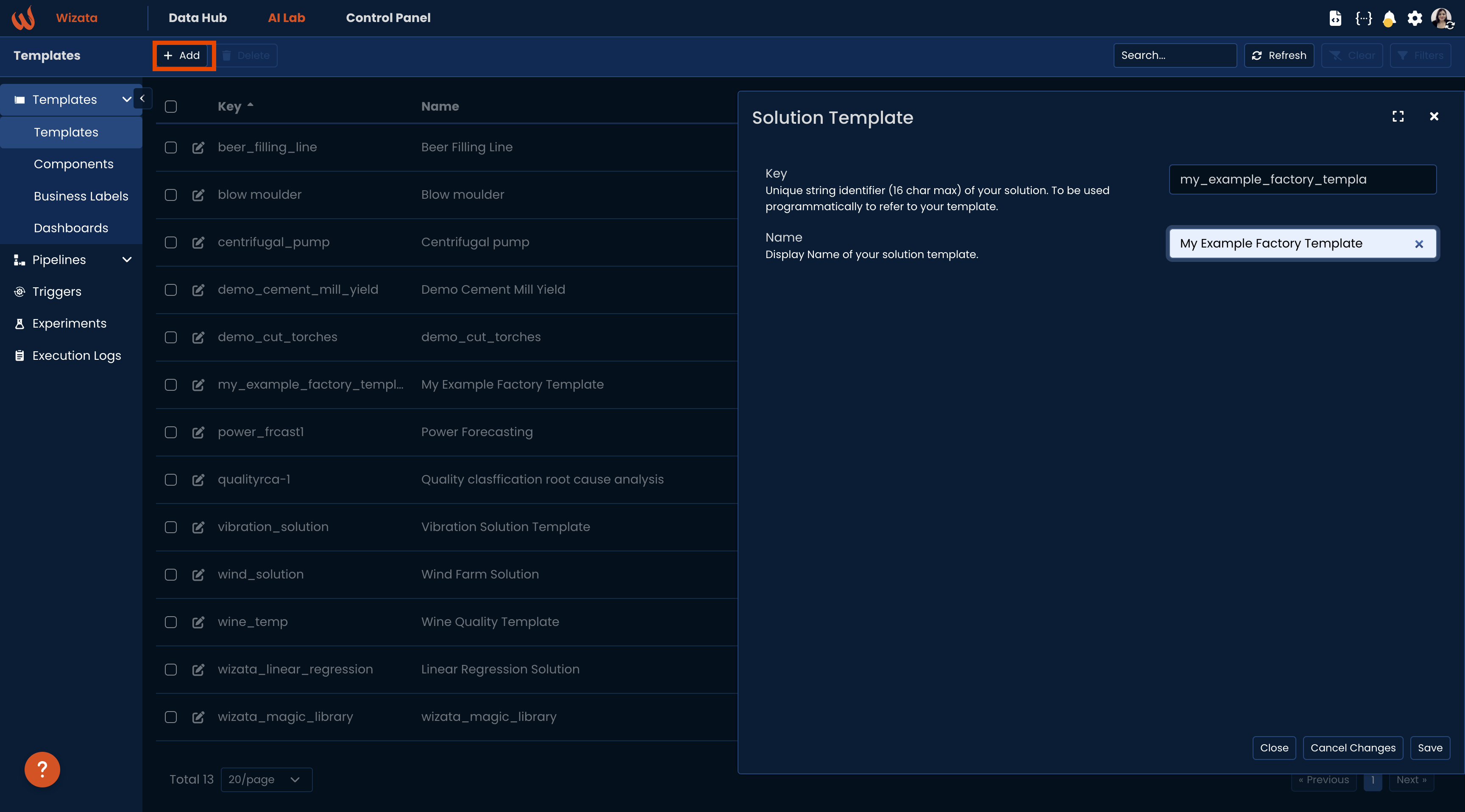
Image 1: Creating a template
With the template_key you can also use lists
and get
methods linked to templates:
my_template = wizata_dsapi.api().get(template_key='my_example_factory_template')
Additionally, you can delete a template property using the remove_property()
method, passing the template property name
as a parameter:
# Removing humidity as a template property
wizata_dsapi.api().remove_property(name='Bearing1')
Or with the UI, once you click on the Edit Template Properties button inside your specific template:
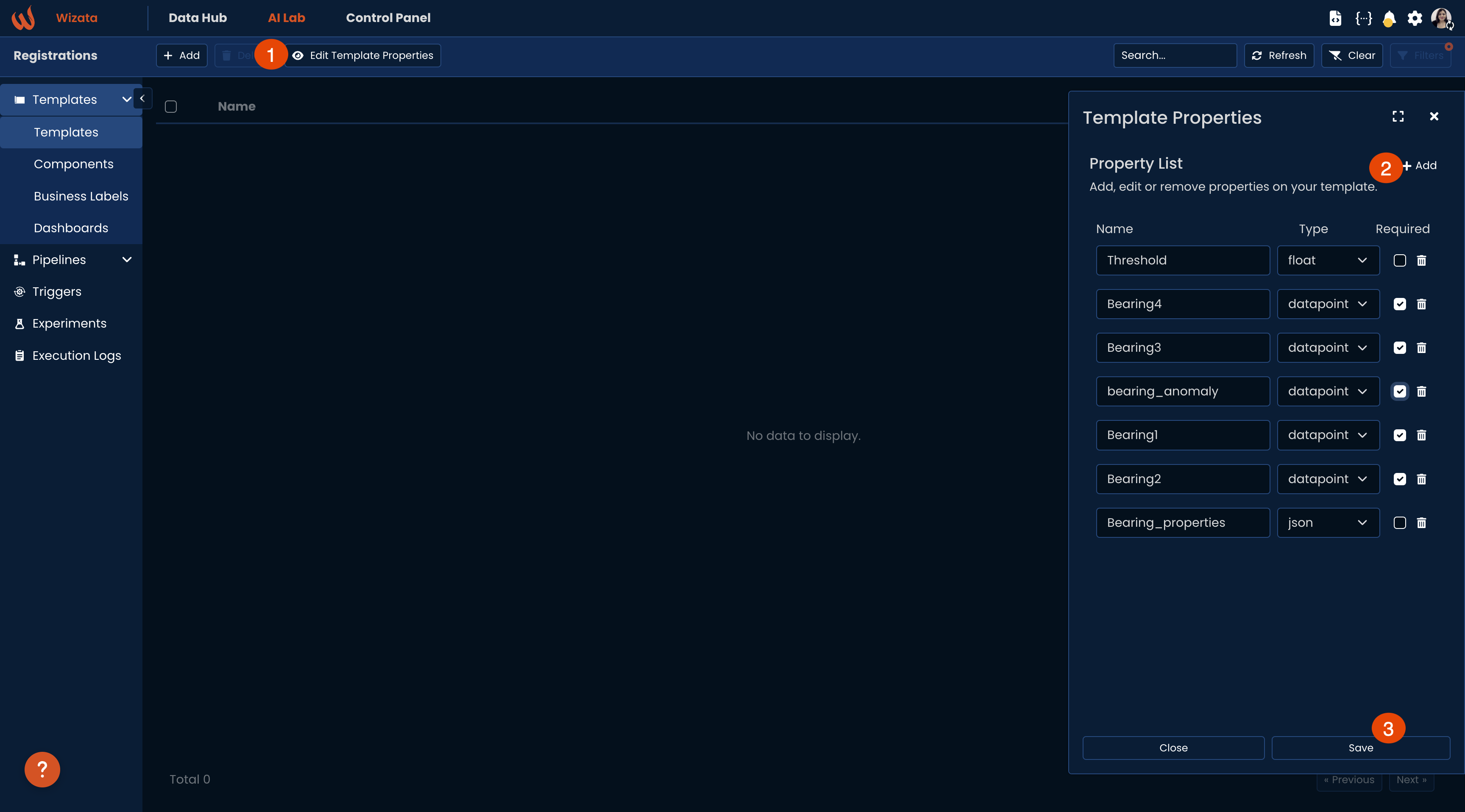
Image 2: Editing Template Properties
Property type
A property can have multiple types:
- datapoint: str corresponding to the hardware ID of datapoint.
- JSON: Can be any complex JSON object, passed as a dict in python (must be serializable)
- string: Char chain of type str.
- integer: Is an int.
- float: Is a floating decimal value.
- relative: Is a time format based on now value; Format is now, then '+' or '-', then an integer value, then a unit ( y, M, d, h, m, s, ms. For example: “now-1d” , “now+1h”, “now”, “now-2M”, …)
- datetime: Is a fixed datetime specified as an integer representing a ms epoch. All datetime are UTC based.
At the exception of datapoint when being used in a pipeline, all properties are passed in variables and context.properties
.
Register a Twin
Once your template is created you need to register a Digital Twin. Each asset will have fixed value for variable and/or mapped datapoints.
Therefore, once querying a registered Twin on a template, you don’t need to know the detailed datapoint.
To register the Twin, you will have to create a map between a template property name and the value:
wizata_dsapi.api().register_twin(
template="my_example_factory_template",
twin="motor_1",
properties={
"Bearing1": "mt1_bearing1",
"Bearing2": "mt1_bearing2"
"Bearing3": "mt1_bearing3"
"Bearing4": "mt1_bearing4",
"threshold": 3.1,
"Bearing_properties": {"bearing_pressure_threshold": 89.5,
"bearing_temperature_threshold": 67}
}
)
Once you register a twin, you need to set all properties correctly. The API will validate that your registration is respected, but if the template is modified, you will have to manually update all registrations.
Or with the UI, you can easily map the properties and the datapoints:
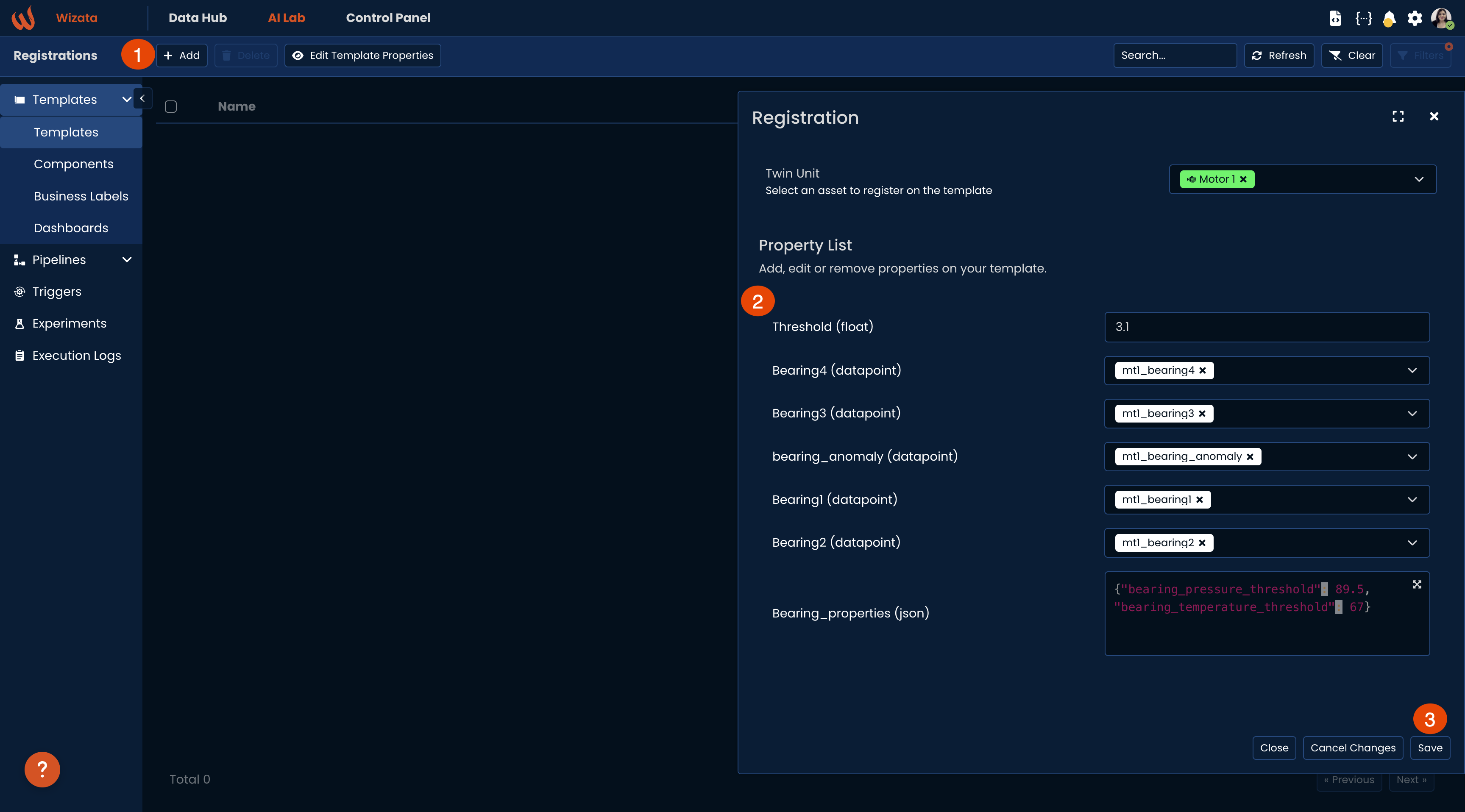
Image 3: Register a Twin
Query Template
After registering a twin on a template, you can Query a dataframe directly based on it:
df = wizata_dsapi.api().query(
template="my_example_factory_template",
twin="motor_1",
datapoints = ["Bearing1","Bearing2"],
start="now-1h",
end="now",
interval=60000
)
The resulting dataframe will use the template property names as column headers.
Any solution based on this dataframe should be compatible with any asset registered on the template.
For example, executing this sample query will return a dataframe with Bearing1
and Bearing2
datapoints as columns headers, as they were previously registered:
Timestamp | Bearing1 | Bearing2 |
---|---|---|
2024-12-30 15:41:33+00:00 | 0.147364 | 0.108148 |
2024-12-30 15:42:00+00:00 | 0.153377 | 0.108274 |
... | ... | ... |
2024-12-30 16:41:00+00:00 | 0.000702 | 0.000269 |
Additionally, you can create templated queries without specifying a detailed datapoints
list.
query = wizata_dsapi.api().query(
template="my_example_factory_template",
twin="motor_1",
start="now-1h",
end="now",
interval=60000
)
print(query)
This query will return a Data Frame with all the previously mapped datapoints as columns headers, for the specific twin (motor_1
) on the selected template:
Timestamp | Bearing1 | Bearing2 | Bearing3 | Bearing4 | bearing_anomaly |
---|---|---|---|---|---|
2024-12-30 15:52:00+00:00 | 0.170515 | 0.105329 | 0.112825 | 0.075072 | 1.0 |
2024-12-30 15:53:00+00:00 | 0.161016 | 0.097687 | 0.105633 | 0.073442 | 1.0 |
... | ... | ... | ... | ... | ... |
2024-12-30 16:19:00+00:00 | 0.220141 | 0.096566 | 0.095933 | 0.076060 | 1.0 |
Deleting a template with its registrations
Before deleting a template, you must first remove all twin registrations associated with it.
You can do this programmatically by using a script to retrieve and delete all the registrations via .get_registrations()
, and then proceed to delete the template itself:
template_object = wizata_dsapi.api().get(template_key="my_example_factory_template")
registrations = wizata_dsapi.api().get_registrations(template_object)
for registration in registrations:
wizata_dsapi.api().delete(registration)
wizata_dsapi.api().delete(template_object)
Alternatively, you can perform the same operation through the UI by first deleting all twin registrations linked to your template:
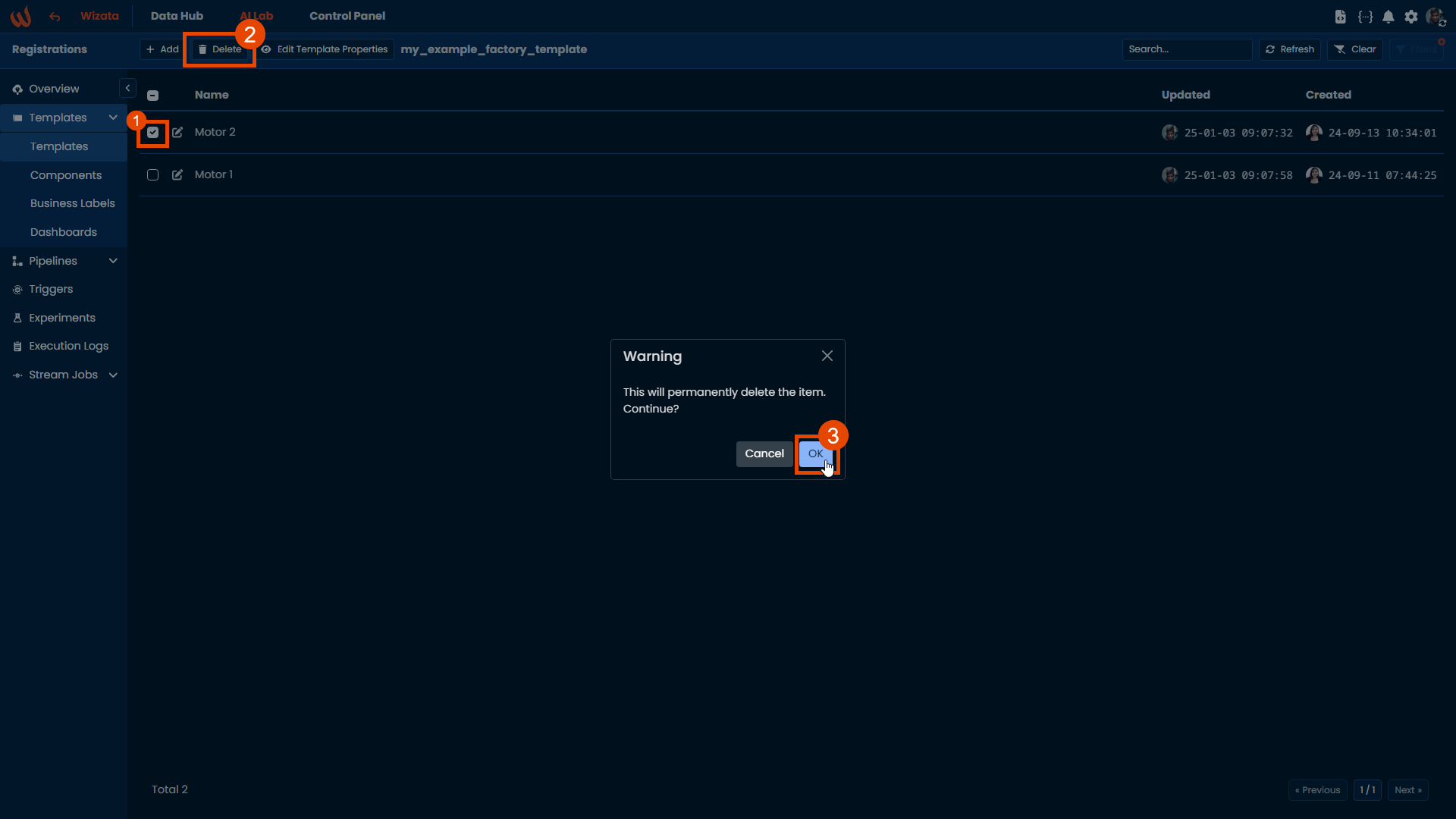
Once all registrations are removed, you can proceed to delete the template itself from the same interface.
Updated about 2 months ago